Contents
This short tutorial will introduce some concepts of the JavaGeom Library. Basic shapes will be created, displayed, and intersected. They will be combined to create new shapes.
Create shapes
Basically, the library manipulates shapes, which are subclasses of the java Shape interface. Most shapes can be created by using direct constructors. For example points can be created directly by giving their coordinates, lines can be created through 2 points or by using static methods in the StraightLine2D class, circles are usually created from a point (the center) and a radius.
// Create some points
Point2D p1 = new Point2D(40, 30);
Point2D p2 = new Point2D(180, 120);
Point2D p3 = new Point2D(20, 120);
Point2D p4 = new Point2D(40, 140);
// Create a circle
Circle2D circle1 = new Circle2D(80, 120, 20);
// Create an edge
LineSegment2D edge1 = new LineSegment2D(p3, p4);
// Create some lines
StraightLine2D line1 = new StraightLine2D(p1, p2);
StraightLine2D line2 = StraightLine2D.createMedian2D(p3, p4);
Display
In the current version of the library, each shape in JavaGeom implements the java.awt.Shape interface. Most of them can be drawn directly to the current graphics object. Points are usually represented by circles to make them more visible.
// The graphics to draw on
Graphics2D g2 = (Graphics2D) g;
// Draw the points
p1.draw(g2);
p2.draw(g2);
// Draw some shapes
circle1.draw(g2);
edge1.draw(g2);
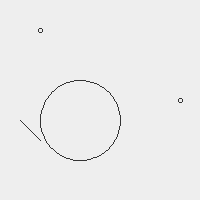
Straight lines can not be drawn directly, as they are unbounded. The
method clip
make them drawable.
The argument is a bounding box, which
contains the max coordinates of the window in each direction.
//The clipping box
Box2D box = new Box2D(0, 200, 0, 200);
// Draw the lines
line1.clip(box).draw(g2);
line2.clip(box).draw(g2)
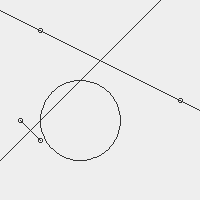
Compute intersections
Straight lines or line segments provide a method to compute the intersection point with another straight object.
Point2D intLine = line2.getIntersection(line1);
intLine.draw(g2);
For other shapes, there can be more than one intersection. All shapes provide a method to compute intersection with a straight line, which returns a collection of Point2D. It is easy to get the intersection points between a line and a circle, for example.
Collection<Point2D> intersects = circle1.getIntersections(line2);
for(Point2D point : intersects)
point.draw(g2);
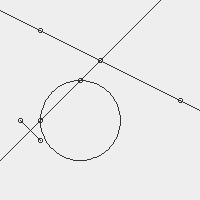
Transforms
Affine transforms allow easy transformation of the shapes. Transforms can be created using static methods in AffineTransform2D class, in a way similar to the java.awt.geom.AffineTransform class.
AffineTransform2D sca = AffineTransform2D.createScaling(p4, 2, .8);
AffineTransform2D tra = AffineTransform2D.createTranslation(30, 40);
AffineTransform2D rot = AffineTransform2D.createRotation(p4, Math.PI/2);
Each shape implements a 'transform' method, which returns the transformed shape. The type of the shape is computed automatically. Straight lines are still unbounded after transform, and need to be clipped.
circle1.transform(tra).clip(box).draw(g2);
line2.transform(rot).clip(box).draw(g2);
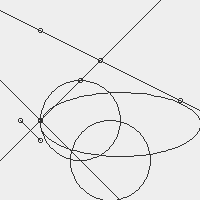
Source code
The complete source code of the tutorial can be downloaded.